A month of Flutter: Stream transforms and failing tests
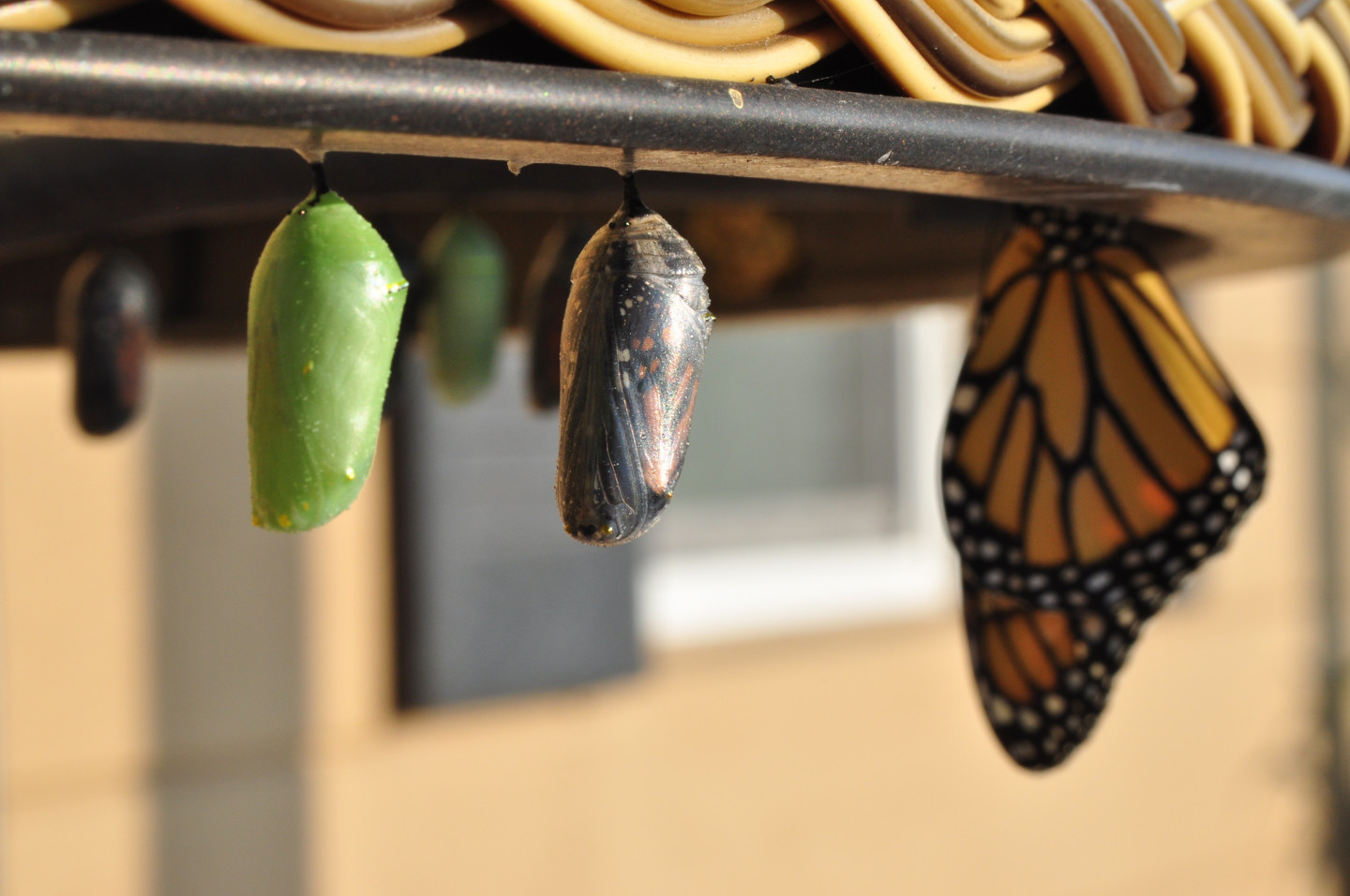
Now that I've got a Post model with mock data and a StreamBuilder for rendering, it all needs to be wired together.
The main changes I'm making are in _MyHomePageState
where I'm replacing the stream of int
s with a call to the new _loadPosts
method.
Stream<List<Post>> _loadPosts(BuildContext context) {
return DefaultAssetBundle.of(context)
.loadString('assets/posts.json')
.then<List<dynamic>>((String value) => json.decode(value))
.asStream()
.map(_convertToPosts);
}
_loadPosts
uses DefaultAssetBundle.of
to get the most appropriate AssetBundle
for loading files from the assets
directory.
Reading the String
contents of a file is asynchronous, after which the JSON contents can be decoded. The loading and decoding will happen as a Future
but I want to be working on a stream so I'll use asStream
to convert the Future
to a Stream
.
Finally the Stream will then get transformed using map
and another new method _convertToPosts
.
List<Post> _convertToPosts(List<dynamic> data) {
return data.map((dynamic item) => Post.fromMap(item)).toList();
}
Every time an event comes down the stream, map
will call the convert method on the value. In this case the value is a List
of items and List
's map
will pass each item to Post.fromMap
. Note that map
on a List
is different from map
on a Stream
. map
on a List
is lazy so we'll force it to execute with a final toList
.
The rest of the changes are going thought the code base and updating List<int>
types to be List<Post>
types and passing Post
instances into the rendering PostItem
s. I'll also replace the hard coded 'Prim Birb'
text with the dynamic username
from the Post
instances.
As I was updating the tests to use the new mock JSON data, I kept running into failures. I was seeing tests timing out or the PostsList
error handling showing errors instead of content. After some digging it turns out that the code in testWidgets
can't access files or assets. With CI configured I can't merge code without passing tests so come back tomorrow to see how I fix them.