A month of Flutter: no content widget
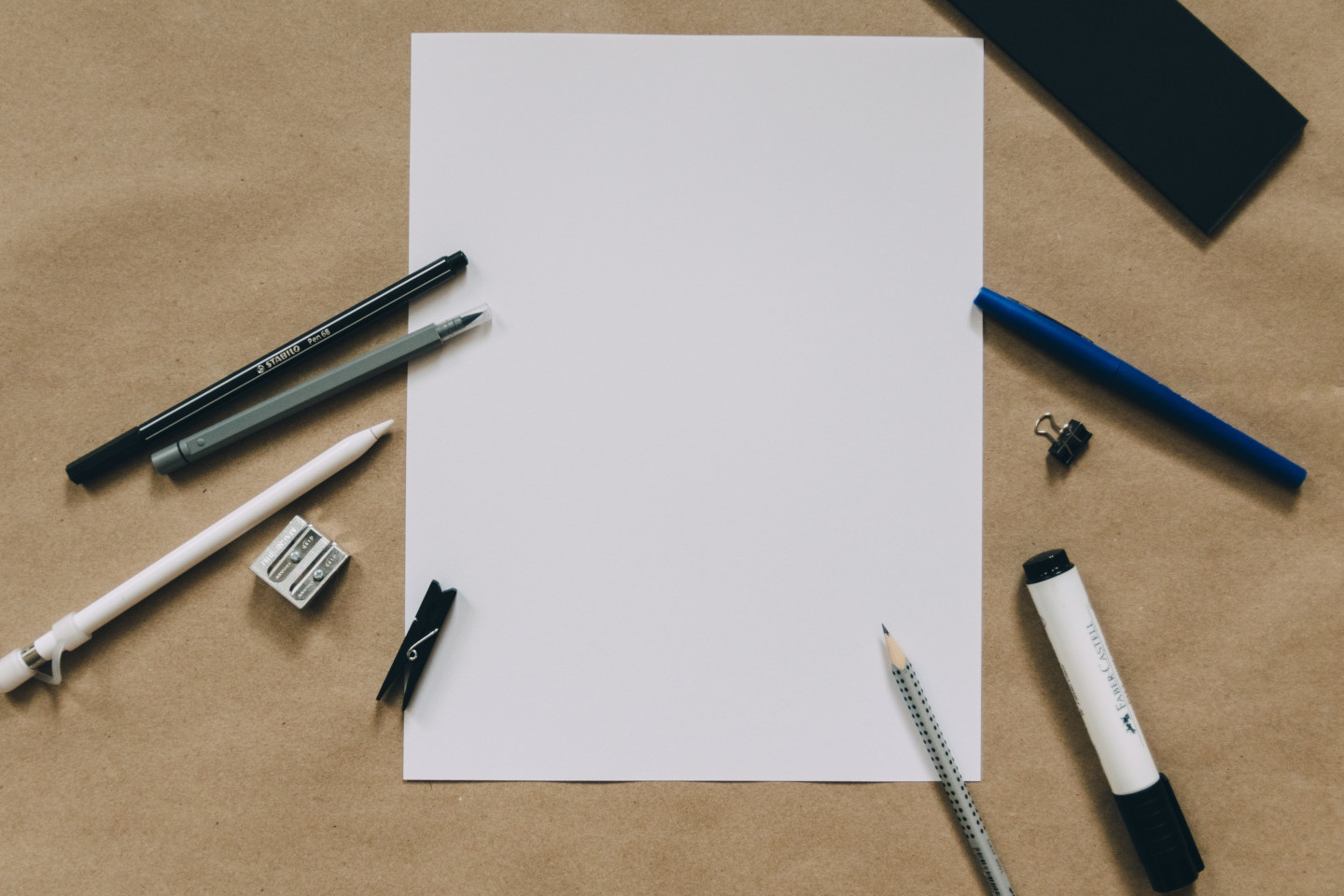
With any sort of content site, there is always a chance of having nothing to display. So let's create a NoContent
widget to use in that scenario.
For this view I'm using an illustration from the amazing unDraw site. I've choosen the SVG version as it's small, scales to any device size, and is easy to change the color of if themes are implemented.
Since it's an SVG, it'll require the flutter_svg package to render. Packages are easy enough to add as dependencies to your app. The SVG file will also need to be added to an assets directory and included in pubspec.yaml
.
Then a new file, no_content.dart
, will be created with the following StatelessWidget
definition:
class NoContent extends StatelessWidget {
const NoContent();
@override
Widget build(BuildContext context) {
return Container(
width: MediaQuery.of(context).size.width,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SvgPicture.asset(
'assets/undraw_a_day_at_the_park_owg1.svg',
height: 300.0,
),
const Text('No Birbs a birbing'),
],
),
);
}
}
The main goal here is to render the SVG with some text underneath it. Since nothing in NoContent
is going to change, let's give it a const
constructor. We'll also make it so the Column
contents are center aligned vertically and that the Container
takes up the entire view width.
With NoContent
implemented, we'll just swap it into the Scaffold
body for rendering and update the tests accordingly.
Here is the result. The size and spacing of everything will have to be adjusted as the app design evolves but this is a good base implementation.