A month of Flutter: mocking Firebase Auth in tests
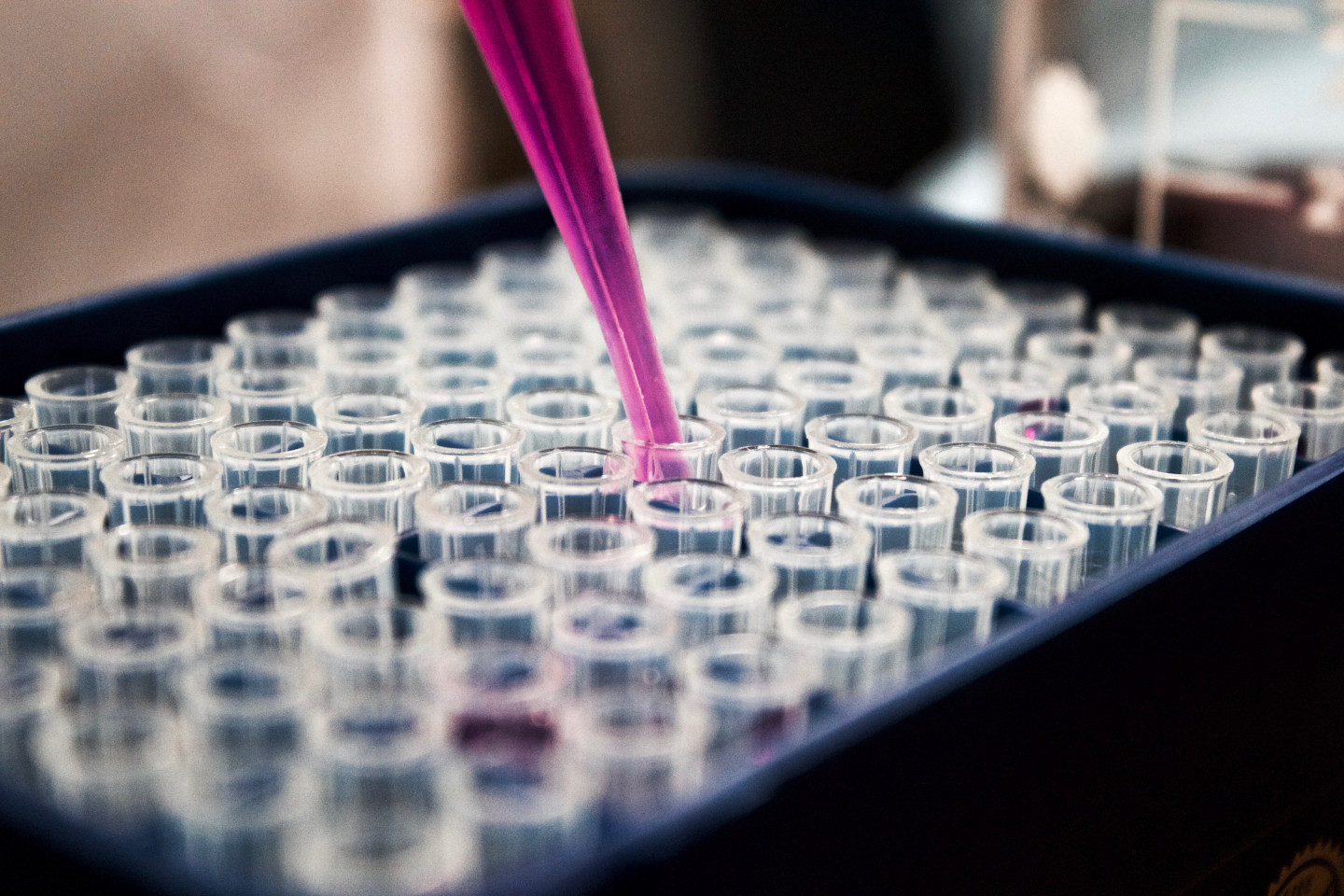
Sign in with Google adds a wildcard to the app: external services. External services are usually avoided in test environments because they create variance and complexity so today I'm going to talk about creating mocks to simulate interacting with these third-party APIs.
Mocks in tests provide two main features: stubbing methods and objects so they behave like real things and validation that methods and properties are called as expected.
To create a mock for FirebaseAuth
is as simple as:
class FirebaseAuthMock extends Mock implements FirebaseAuth {}
I've also created FirebaseUserMock
, GoogleSignInMock
, GoogleSignInAuthenticationMock
, and GoogleSignInAccountMock
. For now they are mostly simple mocks but they can be created with more complex states and responses stubbed.
The majority of the tests written for yesterday was testing the Auth
service.
group('Auth', () {
final FirebaseAuthMock firebaseAuthMock = FirebaseAuthMock();
final GoogleSignInMock googleSignInMock = GoogleSignInMock();
final FirebaseUserMock firebaseUserMock = FirebaseUserMock();
final GoogleSignInAccountMock googleSignInAccountMock =
GoogleSignInAccountMock();
final GoogleSignInAuthenticationMock googleSignInAuthenticationMock =
GoogleSignInAuthenticationMock();
final Auth auth = Auth(
firebaseAuth: firebaseAuthMock,
googleSignIn: googleSignInMock,
);
test('signInWithGoogle returns a user', () async {
when(googleSignInMock.signIn()).thenAnswer((_) =>
Future<GoogleSignInAccountMock>.value(googleSignInAccountMock));
when(googleSignInAccountMock.authentication).thenAnswer((_) =>
Future<GoogleSignInAuthenticationMock>.value(
googleSignInAuthenticationMock));
when(firebaseAuthMock.signInWithGoogle(
idToken: googleSignInAuthenticationMock.idToken,
accessToken: googleSignInAuthenticationMock.accessToken,
)).thenAnswer((_) => Future<FirebaseUserMock>.value(firebaseUserMock));
expect(await auth.signInWithGoogle(), firebaseUserMock);
verify(googleSignInMock.signIn()).called(1);
verify(googleSignInAccountMock.authentication).called(1);
verify(firebaseAuthMock.signInWithGoogle(
idToken: googleSignInAuthenticationMock.idToken,
accessToken: googleSignInAuthenticationMock.accessToken,
)).called(1);
});
});
First I'm instantiating five mock objects and an instance of Auth
which is getting passed the FirebaseAuth
and GoogleSignIn
mocks. These are the primary mocks that represent those two services in the functional code.
Within the test
example I'm specifying that when signIn
gets called on the GoogleSignIn
mock, it should return an instance of the GoogleSignInAccount
mock using thenAnswer
. thenAnswer
is just like thenReturn
but is for returning asynchronous values.
There are then stubs for two more external services before the actual signInWithGoogle
method being tested. This expect
asserts the response is the mocked FirebaseUser
that it should be.
After that I have three verify
assertions. These check with the mocks to make sure the API methods were called with the expected inputs. With this test in place I can be reasonably sure that as long as the external services don't change their APIs, my code will keep working as desired.
The Auth
tests need to be fleshed out some to make sure they handle error cases in the external services. There was an issue created to track adding this error handling.
The last part I want to test is that when a user taps on the Sign in with Google FAB, the Auth
service will be called as expected. The pattern of mocks here is the same as above but just simpler.
testWidgets('Renders sign in', (WidgetTester tester) async {
final AuthMock mock = AuthMock();
// Build our app and trigger a frame.
await tester.pumpWidget(MaterialApp(
home: SignInFab(auth: mock),
));
when(mock.signInWithGoogle())
.thenAnswer((_) => Future<FirebaseUserMock>.value(FirebaseUserMock()));
expect(find.byType(Image), findsOneWidget);
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(find.text('Sign in with Google'), findsOneWidget);
await tester.tap(find.byType(FloatingActionButton));
verify(mock.signInWithGoogle()).called(1);
});
Testing is a critical aspect of making sure applications continue to work as they are changed. These are unit tests and widget tests which are important but there is a third type of test: integration. Integration tests are really good for making sure a full feature flow continues to work. I'll be working on adding integration tests in the future.