A month of Flutter: Firestore create user rules and tests
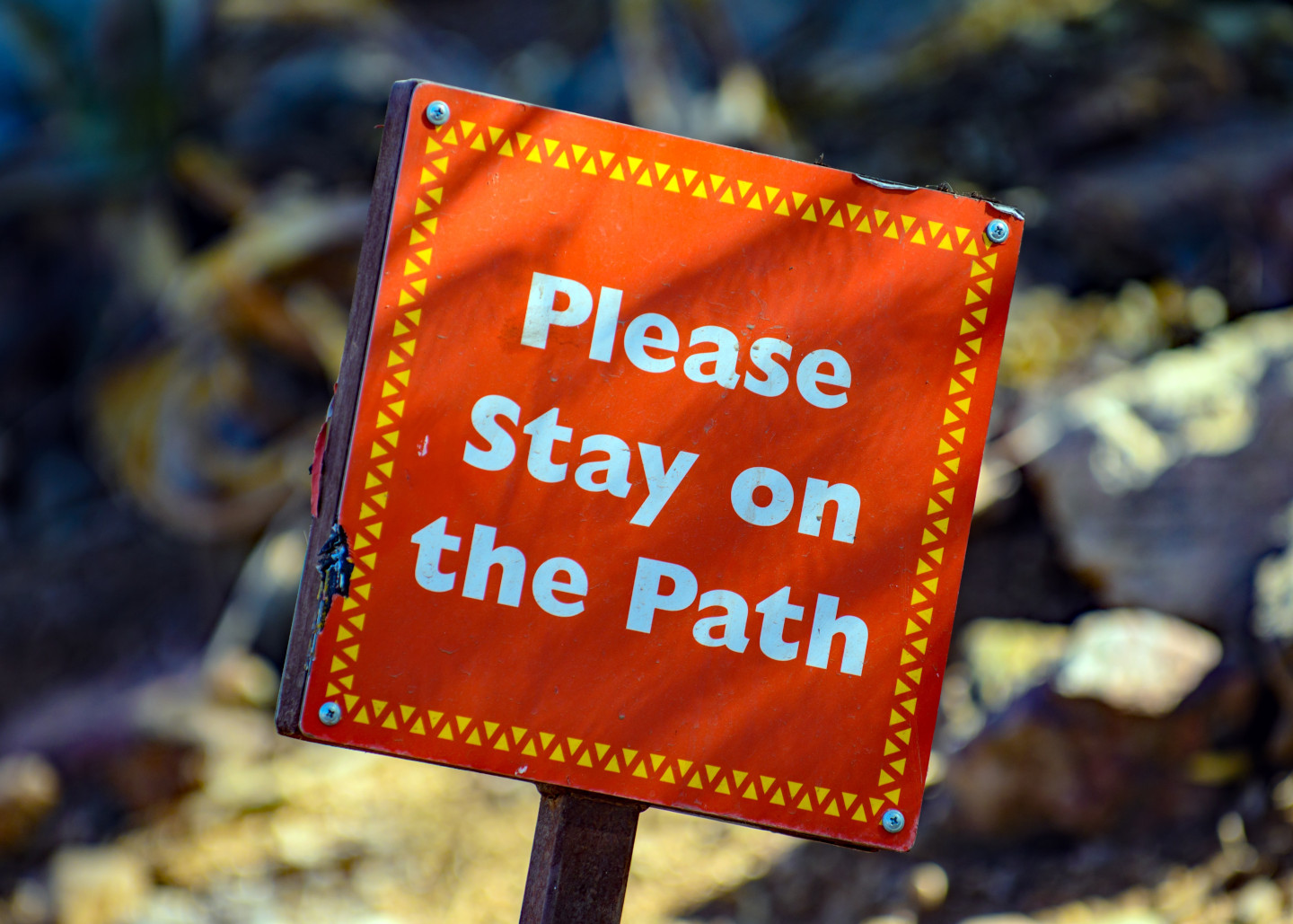
When a user signs in with Google I'm going to create a user document in Firestore. Each authenticated user should only be able to create one user document. These documents will eventually be readable by other users so Firestore needs to have server(less)-side validation to keep the data as correct as possible.
Right now I'm working on registering users so I'm only going to implement create
rules, not read
, update
, etc.
match /users/{userId} {
allow create: if isOwner(userId) &&
// TODO: enable after bug fix https://github.com/firebase/firebase-tools/issues/1073
// validCreateTimestamps() &&
validCreateUser();
}
This will allow user
documents to be created if the owner has the same ID and the document being created passes validation.
Validation of timestamps is being skipped because of a bug in the emulator that causes tests to hang. I've also been considering moving createdAt
and updatedAt
timestamps into Cloud Functions but I'm not ready to make that commitment yet.
User document creation is primarily validated with two tests. One to make sure an authenticated user can create their own document and one to make sure a user can't create a document for someone else.
@test
async 'can create self'() {
const uid = this.user().uid;
const user = this.db({ uid }).collection('users').doc(uid);
await firebase.assertSucceeds(user.set(this.validUser));
}
@test
async 'can not create someone else'() {
const user = this.db(this.user()).collection('users').doc(uuid.v4());
await firebase.assertFails(user.set(this.validUser));
}
Additionally, there are several tests that iterate over a number of invalid values and assert they fail.
There are a number of helper methods I've defined in firestore.rules
:
function isOwner(userId) {
return request.auth != null &&
request.auth.uid == userId;
}
The isOwner
helper checks to see if the user document ID matches that of the current authenticated user.
function validString(key) {
return data()[key].trim() == data()[key] &&
data()[key].size() > 0;
}
The validString
helper checks to see that a required string has a value.
function validUrl(url) {
return url.matches('https://[a-zA-Z].+');
}
The validUrl
helper checks that a string starts with https://
. I'm pretty sure this will not correctly validate some URLs but this value should generally be set to a Google CDN so I don't think invalid hosts will come up.
function validCreateTimestamps() {
return data().updatedAt == request.time &&
data().createdAt == request.time;
}
validCreateTimestamps
checks that the updatedAt
and createdAt
values match the time on the request. This works because the client will set that value with a constant that Firestore will replace with the current time.
function validUser() {
// TODO: prevent extra fields
return validString('nickname') &&
validString('fullName') &&
validString('photoUrl') &&
validUrl(data().photoUrl);
}
function validCreateUser() {
return validUser() &&
data().agreedToTermsAt == request.time;
}
The final two helpers are to validate a user document leveraging all the other helpers. One TODO
I've left for the future is to make sure all fields on the document are on an allowed list.
I'm not completely happy with how the server
tests are currently organized. I will probably do some cleanup in the future to try and make everything more elegant. I plan on adding faker.js for fun too.