A month of Flutter: a list of posts
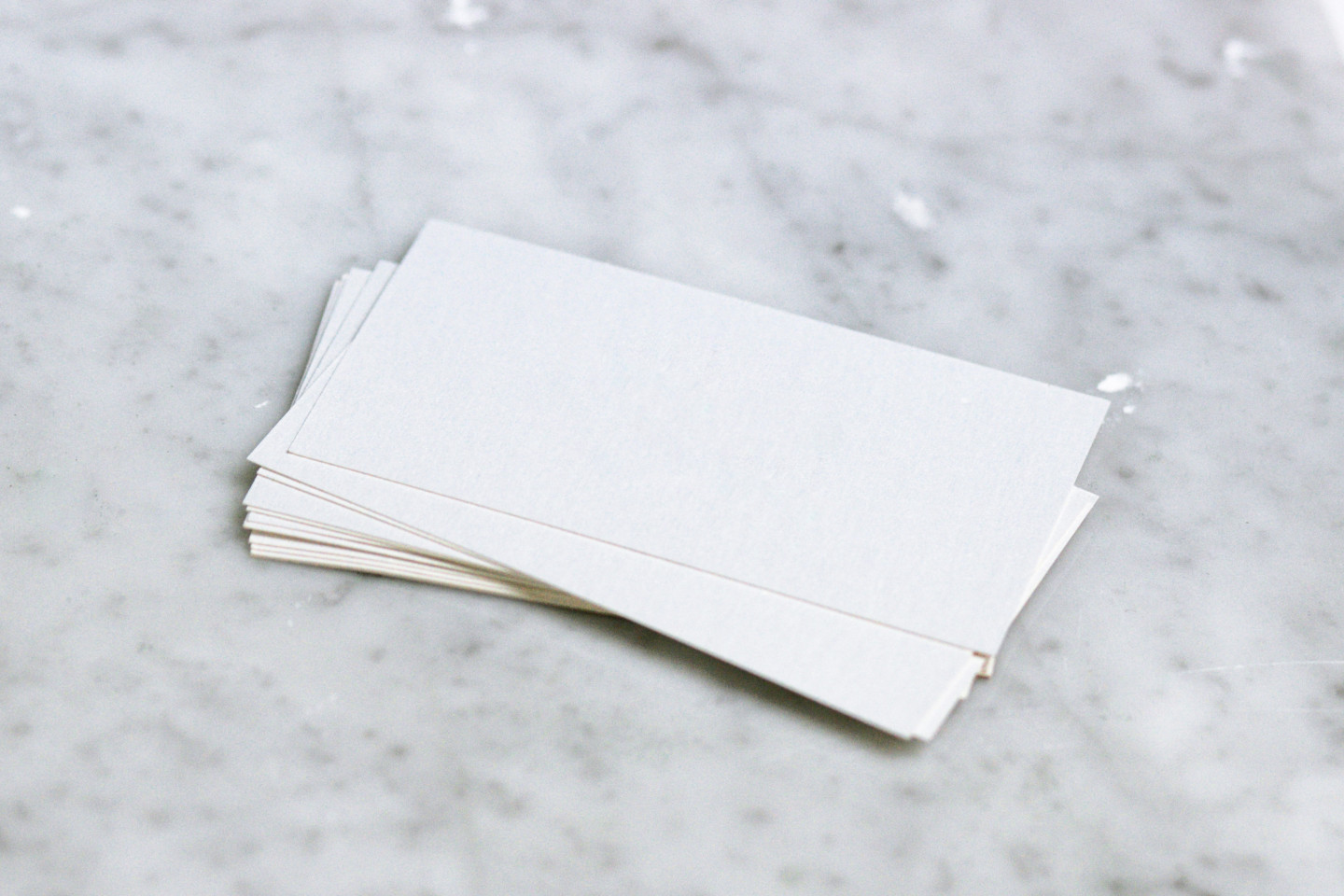
Yesterday I created a widget to display when there are no images available. Today I'm going to create the PostsList
widget that will be displayed when there are images.
The widget will use a ListView
, in part because I plan to use Firebase Cloud Firestore as a datastore and that's the pattern cloud_firestore uses.
For now I'm going to replace the hardcoded NoContent
widget with the new PostsList
.
NoContent
will temporarily go unused until #13 load sample data and render homepage list items is implemented.
For this initial list I'm going to iterate over a finite list and create a static Material Card
for each list item. The card will have a fixed height and some simple Text
.
class PostsList extends StatelessWidget {
const PostsList();
static const List<int> _items = <int>[0, 1, 2];
@override
Widget build(BuildContext context) {
return ListView(
children: _items.map((int index) {
return Card(
child: Container(
height: 300.0,
child: const Center(
child: Text('Prim Birb'),
),
),
);
}).toList());
}
}
I'll also create a simple test to make sure that PostsList
renders two Card
widgets and the expected text. You might notice that the test checks for two of each while the _items
implementation should actually render three cards. But the screen size is not large enough to display all three cards so one of them is offscreen. When testing I only care about what's rendered to the user.
testWidgets('Renders list of posts', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(const MaterialApp(
home: PostsList(),
));
expect(find.byType(Card), findsNWidgets(2));
expect(find.text('Prim Birb'), findsNWidgets(2));
});
The result is a simple list of cards: